Floor Division Javascript
Function Floor #
floor([3.2, 3.8, -4.7]) // returns Array[3, 3, -5] math. floor([3.21, 3.82, -4.71], 1) // returns Array[3.2, 3.8, -4.8] math.Javascript, Using Math.Floor To Round A Number
console . log (Math. log (Math. log (Math. console . log (Math. log (Math. console . log (Math. log (Math. console . log (Math. log (Math. log (Math. console . log ( Math. log (Math. log (Math. console . log (Math. log (Math.# Video | Floor Division Javascript
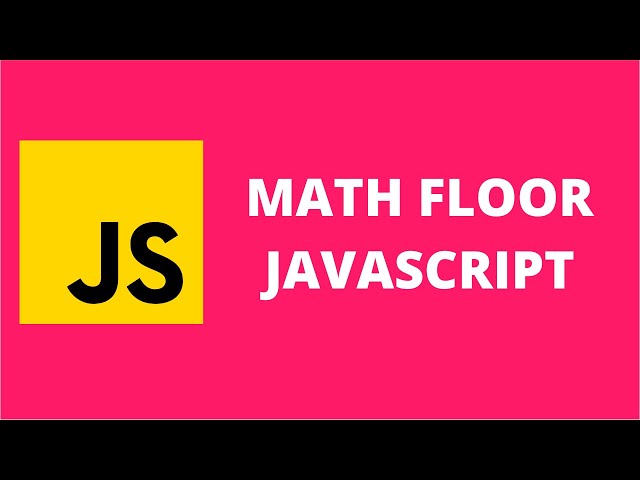
- Javascript Modulo
- Javascript Round
- Math.Floor Javascript
- Javascript Math.Floor 2 Decimal
- Javascript Variable In Integer
Floor Divide Javascript
What Does // Mean In Python? Operators In Python
The only difference is that instead of a single slash / , you use a double slash // :firstNum // secondNum
Examples of floor division
In the example below, dividing the ground 12 by 5 resulted in 2:
num1 = 12 num2 = 5 num3 = num1 // num2 print("floor division of", num1, "by", num2, "=", num3) # Output: floor division of 12 by 5 = 2
Whereas the regular division of 12 by 5 would equal 2.4. Let 2 remain 4:
num2 = 5 num3 = num1 / num2 print("normal division of", num1, "by", num2, "=", num3) # Output: normal division of 12 by 5 = 2.4
This shows you that the // operator rounds the result of dividing two numbers to the nearest whole number. num1 = 29 num2 = 10 num3 = num1 / num2 num4 = num1 // num2 print("normal division of", num1, "by", num2, "=", num3) print("but ground division of", num1 , "by", num2, "=", num4) """ Output: normal division of 29 by 10 = 2.9 but ground division of 29 by 10 = 2 """
And if you perform a ground division with a negative number, the result will always be rounded down. num1 = -12 num2 = 5 num3 = num1 // num2 print("floor division of", num1, "by", num2, "=", num3) # floor division of -12 by 5 = -3
The Double Slash // operator works like math.floor()
In Python, math.floor() rounds a number to the nearest integer, just like the double slash // operator does. Here is an example :
import math num1 = 12 num2 = 5 num3 = num1 // num2 num4 = math.floor(num1 / num2) print("floor division of", num1, "by", num2, "=", num3) print("math .floor of", num1, "divided by", num2, "=", num4) """ Output: floor division of 12 by 5 = 2 math.floor of 12 divided by 5 = 2 """
You can see that math.floor() does the same thing as the // operator. You can also use this __floordiv__() method directly instead of the // operator:
num1 = 12 num2 = 5 num3 = num1 // num2 num4 = num1.__floordiv__(num2) print("floor division of", num1, "by", num2, "=", num3) print("using floordiv method gets us the same value of", num4) """ Output: dividing the floor of 12 by 5 = 2 using the floordiv method gives us the same value of 2 """
Conclusion
In this article, you learned how to use the double slash operator // and how it works behind the scenes.
Floor Div Javascript
Tf.Math.Floordiv
tf.compat.v1.floordiv , tf.compat.v1.math.floordivtf.math.floordiv( x, y, name=None )
Mathematically, this is equivalent to floor(x / y). For example: floor(8.4 / 4.0) = floor(2.1) = 2.0 floor(-8.4 / 4.0) = floor(-2.1) = -3.0 This is equivalent to the '//' operator in Python 3.0 and above.
Integer Division Javascript
Integer Division
For example, while "normal" or floating point division of 1/4 yields 0.25, integer division of this same expression yields zero. Similarly, 26/5 will give 5 in integer division, but 5.2 in floating point division. If both or either operands are floating-point types (float, double, or decimal), the / operator results in floating-point division. var integerDivision = 26 / 5; var floatDivision = 26 / 5.0;Also, the result of integer division is always rounded towards zero. it is always truncated:
var integerDivision = - 26 / 5;
In other languages? Java
The division rules in Java are the same as in C#:
var integerDivision = 26 / 5; var negativeIntegerDivision = - 26 / 5; var floatingdivision = 26 / 5.0; var negativeFloatingDivision = - 26 / 5.0;
JavaScript
In JavaScript, the / operator always results in floating point division:
var quotient = 26 / 5; var negative quotient = - 26 / 5;
Python
In Python, the // operator is used as a floor function. This is similar to integer division, but the result is always rounded instead of truncated:
positiveDivisionInteger = 26 // 5 negativeDivisionInteger = - 26 // 5
The / operator in Python will cause floating point division:
positiveFloatDivision = 26 // 5 negativeFloatDivision = - 26 // 5
Why is it important? For me, I encountered integer division because I was trying to calculate an integer percentage via the following:
int part = someNum; int total = someOtherNum; int percentage = 100 * ( part / total )
Since part was smaller than total , the result of the integer division (and it was an integer division because the numerator and denominator were integers) was zero. Reviewing my code resulted in floating point splitting, as I expected:
double part = someNum; double total = someOtherNum; double decimal percent = part / total; int percentage = (int) (100 * decimalPercentage);
I've been coding for several years now, and honestly, I hadn't thought about integer division versus floating point division since my last computer science class a few years ago.
# Images | Floor Division Javascript - Javascript Math.Floor 2 Decimal
Javascript Round - Integer Division
Floor Function - JavaScript, using Math.floor to round a number